CS Theory
C++ Tutorial 1
Introduction
To start with, this tutorial was written with the goal of providing a foundation on which more advanced topics, such as Data Structures And Algorithms, will be discussed. As a result, some topics might not be covered in their entirety with the expectation that they will be discussed in greater depth at a later date. Secondly, this tutorial assumes that you have no background in programming. If you have experience with another programming language feel free to skip around the tutorials since new code will not build upon existing code. New concepts will however build upon existing ideas so skipping is not recommended for beginners. Additionally, this tutorial does not explain how to install or set up your programming environment/IDE/which ever method of learning the language you have in mind. There are simply too many different variables related to setting up such an environment and there are already good tutorials on the internet related to this topic. If you are looking for recommendations or just a starting place than consider the following: if you are an a windows device then either Visual Studio is a good choice; OS X users generally recommend XCode; Linux users generally do not use an IDE but rather use a compiler, such as GCC, along with a text editor and of course a terminal emulator. Code::Blocks is also a viable alternative on each one of the platforms.
C/C++ Similarities And Differences
C is another programming language which predates C++. C++ is based on C and is nearly a perfect super set of C. C is still used and new features are added to the language – but the old version of C which C++ is based on continues to move apart from newer versions of C. Because of this, there are several corner cases which make some C and C++ code incompatible, but for the most part whatever code you might want to write in C will work in C++ with few alterations at the most. But critically, they are two different languages and mastery of one language does not mean imply a mastery of the other. Since C is probably the most influential programming language and since C++ was originally conceived as a series of extensions to the language the similarities between these two are especially strong. In the nature of simplicity, this tutorial will only discuss C++.
Overview
The process which you must remember through this tutorial is the compilation model which translates human readable code into executable assembly language. When a programmer wishes to write a program, they write human readable code called source code. The compiler is a program which takes this code and translates it into assembly language, which is reducible to 0's and 1's, that allows us to execute the code. In totality, there are three steps which must take place after writing the code in order for the a user to run the program.
- Compilation (Compile Time)
- Linking (Linker Time)
- Execution (Run Time)
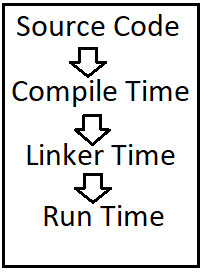
Hello, World!
Long standing tradition dictates that the first program we write is a program that prints out, to whatever standard output happens to be available to your environment, the phrase: "Hello, World!" without quotes. Although it probably does not sound particularly exciting, this program will ensure that you have your environment set up properly. Copy and paste the following code into your environment, run it and then press the "ENTER" key. Afterwards we will go through the code line by line.
using namespace std;
int main()
{
cin.get();
reteurn 0;
The first two lines of code:
using namespace std;
Are required to run the code in order for the compiler to know which functions resources we will be using in the program. It is not essential for you to understand what those two lines of code mean at this time, we will revisit them in the near future.
Likewise, the next two lines of code:
{
Are mainly there for book keeping and don't really matter that much with regards to our program. Similarly, we will be discussing them in greater detail shortly.
The meat of the program begins in the next line
This line tells the computer to print phrase, formally called a "string", "Hello, World!". This is not a useful thing at the moment, however after our programs become more complex the ability to print simple passages of text to standard output will become almost essential.
After that line of code we see the following:
This line of code is actually optional and is really only useful on Windows platforms where the standard output is typically a command prompt, cmd.exe, which appears and disappears too quickly to read. This line of code tells the computer to wait for the user to press "ENTER".
The next two lines of code:
Will not mean very much at this time, and it is technically optional for most compilers, but it will not always be optional so it's a good idea to get into the habit of using it.
That's the end of the first C++ tutorial. In this tutorial, we were able to ensure our compiler was set up correctly by printing the string "Hello, World!" to standard output. It might not sound like a significant set forward, but first steps never seem like that and quite soon we will be exploring much more advanced programs.